When preparing for an interview, knowledge of Advanced Java is essential for standing out in today’s competitive IT landscape. Advanced Java goes beyond the basics and delves into enterprise-level development, server-side applications, frameworks like Spring and Hibernate, and more.
In this blog, we'll explore some of the most frequently asked Advanced Java interview questions and their answers to help you confidently approach your next interview.
- What is JDBC?
JDBC stands for Java Database Connectivity which is used to manipulate and connect for database.
- What is servlet?
Servlet is a taking the request from client and giving the response to the client in other words Servlet is program that run on a Web or Application server and act as a middle layer between a request coming from a Web browser(Client) or other HTTP client and databases or applications on the HTTP server.
- What is JSP?
JSP stands for Java Server Pages and JSP contains both HTML and JSP tags. In JSP tags we use java code like scriptlet tag.
JSP is a server side programming technology is used to develop web applications and to create dynamic web content.
- What is the life cycle of servlet?
In servlet lifecycle contains three stages
Init(): This is used to initialize servelet by calling init() method
Service(): This is used to process the client request
Destroy(): This is used to terminate servlet request
- What are the steps to create JDBC connection?
The following steps to create a JDBC connection
i. Import the packages
ii. Register the drivers
iii. Establish a connection
iv. Create a statement
v. Execute the query
vi. Retrieve results
vii. Close the connections
- When destroy() method of servlet gets called?
The destroy() method is called only once at the end of the life cycle of a servlet.
- What are the different types of statements in JDBC?
Statement, preparedstatement and callable statement are the statements in JDBC
Statement: it is used to access data from database for static SQL statement at runtime and This statement cannot accept parameters.
Prepared statement: it is used to access data from database dynamically and by using parameters to execute SQL Query at runtime
Callable statement: Callable Statements are accepts database stored procedures and if you want access data from database in the form of Stored procedure. It accept input parameters of SQL Query.
- What is the difference between execute(), executeQuery() and executeUpdate()?
execute(): The return type of execute is Boolean if true a resultSet object will be retired otherwise it returns false. This method is used to execute both select and update SQL queries.
Syntax:
Boolean execute(String SQL)
executeQuery(): the return type of executeQuery() is ResultSet and it is used only on select statement of SQL query.
Syntax:
ResultSet executeQuery(String SQL)
executeUpdate(): The return type of executeUpdate() is integer, if it return 0 indicates nothing to execute SQL Query. This method is used for update,delete and insert Sql Queries.
Syntax:
Int executeUpdate(String SQL)
- What is the difference between ServletConfig and ServletContext?
ServletConfig is used for sharing init parameters specific to a servlet while ServletContext interface is for sharing init parameters within any Servlet within a web application.
ServleteConfig object represents unique object per/single servlet and ServletContext complete web application running on JVM with unique object.
getServletConfig() method is used to get the config Object and getServletContext() method is used to get context object.
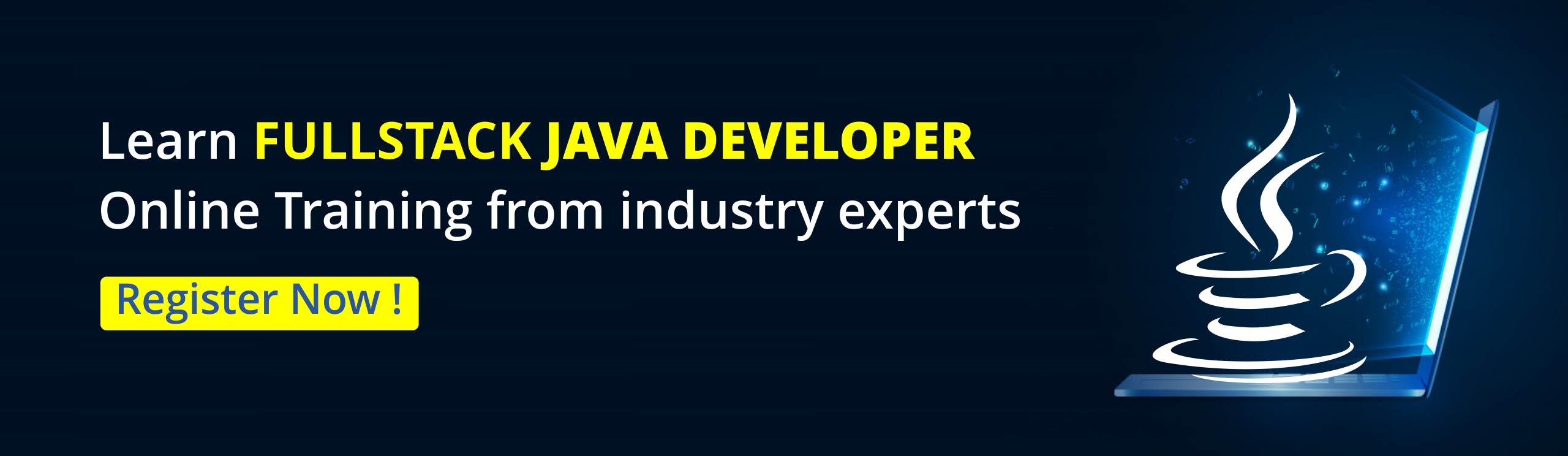
- How to create statement interface in JDBC?
The following procedure to create statement interface in JDBC by using createStatement() method
Statement stmtement = null;
try {
stmtement = conn.createStatement( );
. . .
}
catch (SQLException e) {
. . .
}
finally {
. . .// without close connection
}
- How to create prepared statement interface in JDBC?
The following procedure to create preparedStatement interface in JDBC by using prepareStatement(SQL) method.
PreparedStatement pstmt = null;
try {
String SQL = ""; //SQL Query
pstmt = conn.prepareStatement(SQL);
. . .
}
catch (SQLException e) {
. . .
}
finally {
. . . // without close connection
}
- Can you create a Deadlock condition on a servlet?
Yes, Deadlock situation can be created on a servlet by calling doPost() method inside doGet() method, or by calling a doGet() method inside doPost() method will successfully create a deadlock situation.
Sample Example:
public class TestServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request, response);
response.getWriter().append("Served at: ").append(request.getContextPath());
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
- How to create callable statement interface in JDBC?
The following procedure to create Callable Statement interface in JDBC by using prepareCall(SQL) method.
Connection con = null;
CallableStatement callstmt = null;
try {
String SQL = "{call getEmpName (?, ?)}";
callstmt = con.prepareCall (SQL);
. . .
}
catch (SQLException e) {
. . .
}
finally {
. . .// without close connection
}
- What is the difference between sendredirect and requestdispatcher?
Send Redirect request process is taken care by web container and transfers the request using a browser, each request is processed as a new request. RequestDispatcher request process is taken care by web container, each request is processed without the client being informed.
Using sendRedirect() it doesn't include any resources but using requestDispatcher() it includes the resources.
SendRedirect is client-side process and Request Dispatcher is server-side process.
- How to delete cookie using Serlvet?
The following steps to delete cookie using servlet
i. Read existing cookie and store it in cookie object
ii. Set cookie as zero using setMaxAge() method to delete exist cookie
iii. Add this cookie back into response header
Example:
Cookie cookie=new Cookie("user","");
cookie.setMaxAge(0);
response.addCookie(cookie);//adding cookie in the response
- How to create cookie using Serlvet?
The following steps to create cookie using servlet
i. Create cookie object
ii. Add cookie in response by using addCookie(CookieObject)
Example:
Cookie cookie=new Cookie("user","NareshIT");//create cookie object by using key and value
response.addCookie(cookie);//add cookie in the response
- What is URL Rewriting?
URL rewriting means to add some specific data on the end of URL(Unifom resourse locator) to identify the session in other words it is a automatic process to alter a program for manipulating the parameters in URL.
For example user web browser sends request ‘nareshit.in/all-courses’ so the url is manipulated automatically by server add some data like ‘nareshit.in/all-courses;sessionid=123’ here sessionid=123 is identifier.
- How to read from data in Servlet?
Data From User Interface like JSP to servlet handles automatically based on situation
The following three methods are using servlet read from JSP data
getParameter(): by using request.getParameter() method to read one from UI
getParameterValue(): when call this method like request.getParameterValue() it reads multiple values like booking ticket selection.
getPrameterNames() : when call this method it access list all parameters in current request
- What is Cookie?
A cookie is a piece of information that is present between multiple client requests. A cookie has a name, a single value, and optional attributes such as a comment, path and domain qualifiers, a maximum age, and a version number.
- What are the annotations used in Servlet 3?
The following 3 important annotations are used in the servlets.
@WebServlet : for servlet class.
@WebListener : for listener class.
@WebFilter : for filter class.
- What is the use of RequestDispatcher Interface?
RequestDispatcher interface handle the request from client and dispatches to their resources such as servlet,JSP,HTML. It hides the information to client browser for security and it contains two methods like forward and include.
Syntax:
public void forward(ServletRequest request, ServletResponse response)
Forwards request from one servlet to another resource like servlet, JSP, HTML etc.
public void include(ServletRequest request, ServletResponse response)
Includes the content of the resource such as a servlet, JSP, and HTML in the response.
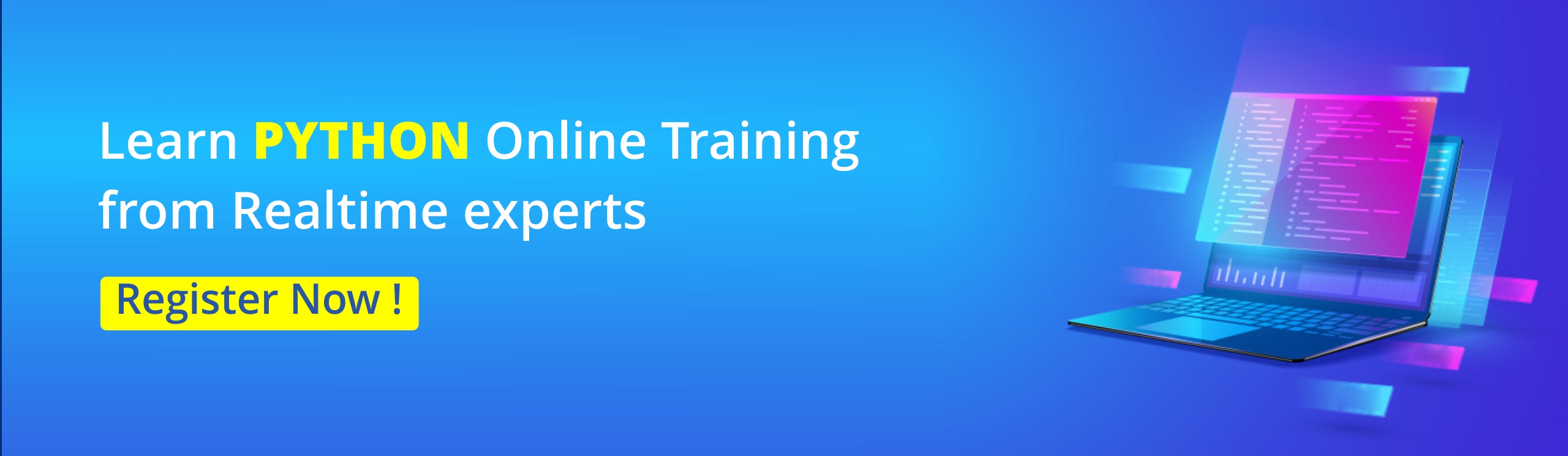
- Why do we use sendredirect() method?
The sendredirect() method is client-side infomation. It is used to redirect the response to another resource like Servlet, JSP, HTML. It does not hide the infomration from URL.
Syntax:
void send Redirect(URL);
response.sendRedirect(URL);
- What are the differences between ResultSet and RowSet?
RowSet Object can be serialized but ResultSet Object cannot be serialized.
RowSet either Connected or disconnected from the database but ResultSet always connected to database.
RowSet using the RowSetProvider.newFactory().createJdb cRowSet() method. and ResultSet using the executeQuery() method.
Result Set Object is a JavaBean object but Result Set object is not a JavaBean object.
- What are the methods used in lifecycle of JSP?
There are three methods used in lifecycle of JSP.
i. public void jspInit()
ii. public void _jspService(ServletRequest request,ServletResponse)throws ServletException,IOException
iii. public void jspDestroy()
- How to disable session in JSP?
The following tag is used to disable session in JSP
<%@ page session="false" %>
- What is MVC?
MVC stands for Model View Controller and this architecture follows View is User Interface that is presentation layer and Controller is Business logic layer and Model is Data access layer to access the data from database.
It follows View to Controller to Model to Database.
- What is the use of <jsp:include> tag?
This tag includes the response from a servlet or JSP page during the request processing phase.
- What is <welcome-file-list> tag?
<welcome-file-list> is a property which defines list of welcome-files in web.xml
If you did not define file name while loading the project in the browser or run on server, the tag <welcome-file-list> is used to define the files that invoked automatically by the server.
By default server looks for welcome file as below
index.html
index.htm
index.jsp
Example:
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.jsp</welcome-file>
<welcome-file>default.htm</welcome-file>
</welcome-file-list>
- What is the use of <servlet-mapping> tag?
<servlet-mapping> is used to associates a URL pattern to a servlet instance. <servlet-mapping> contains two elements like <servlet-name> and <url-pattern> It maps url patterns to servlets. When there is a request from a client, servlet container decides to which application it should forward to.
Example:
<servlet-mapping>
<servlet-name>Name-of-servlet</servlet-name>
<url-pattern>/*</url-pattern>
</servlet-mapping>
- What is <session-config>?
<session-config> is an element available in web.xml file. It contains some models like session-timeout, cookie configuration and tracking mode. Session-config is used to define timeout from the server and as well as configure cookie either http as true for provide security. For tracking mode is Cookie or URL which tracks the information.
Example:
<session-config>
<session-timeout>90</session-timeout>
<cookie-config>
<http-only>true</http-only>
<secure>true</secure>
</cookie-config>
<tracking-mode>COOKIE</tracking-mode>
</session-config>
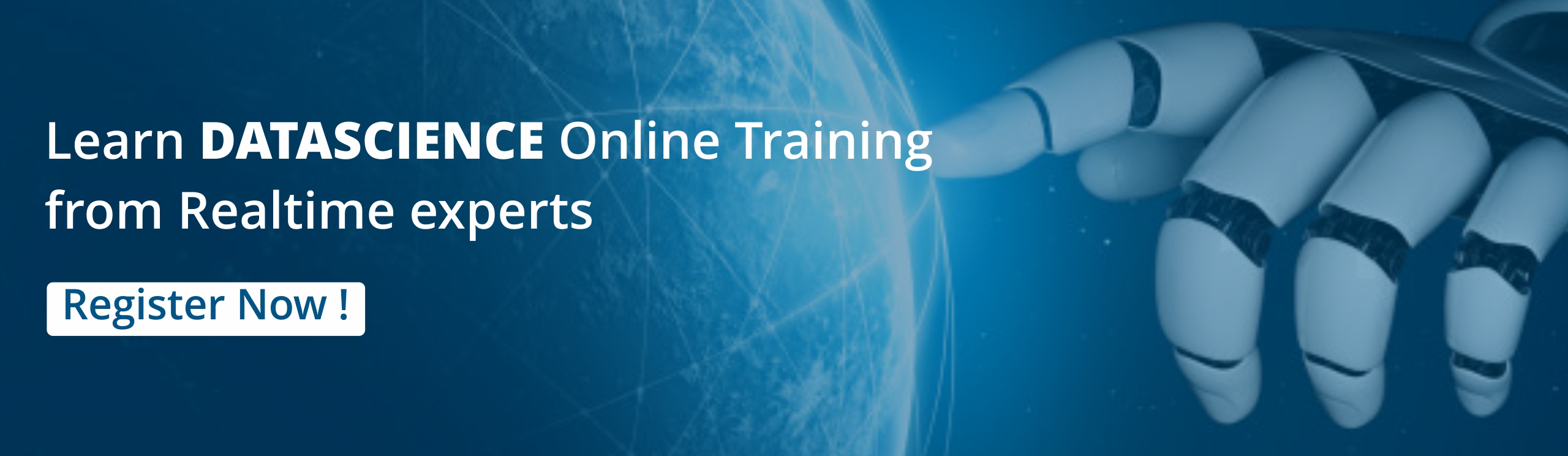
- What is session time out inweb.xml of jsp?
To set a session-timeout that never expires is not desirable because you would be reliable on the user to push the logout-button every time he's finished to prevent your server of too much load (depending on the amount of users and the hardware). Additionaly there are some security issues you might run into you would rather avoid.
The reason why the session gets invalidated while the server is still working on a task is because there is no communication between client-side (users browser) and server-side through e.g. a http-request. Therefore the server can't know about the users state, thinks he's idling and invalidates the session after the time set in your web.xml.
- What is the use of <class-name> tag in web.xml?
You specify the serlvet class name i.e fully quailified java class name and it is mapped to url-pattern by using servlet-name element. This makes it easier to use in the web application, in your html etc. for example com.test.NareshIT here NareshIT is a servlet class and it is fully qualified class name with path.
<servlet-class>com.test.NareshIT</servlet-class>
- What is custom tag in jsp?
Custom tags are known as user defined tags. A tag handler is associated with each tag to implement the operations so, it separates the business logic from JSP and helps avoid the use of scriptlet tags.
Syntax:
without body
<prefix : suffix attribute = “value”/>
with body
<prefix : suffix attribute = “value”>body</prefix : suffix>
We need to follow some steps to create custom tag.
Create the Tag handler class and perform action at the start or at the end of the tag.
Create the Tag Library Descriptor (TLD) file and define tags
Create the JSP file that uses the Custom tag defined in the TLD file
- What is the difference between doGet() and doPost()?
doGet() method is designed to get response context from web resource by sending limited amount of input data, this response contains response header, response body where as doPot() method is designed to send unlimited amount of data along with the request to web resource.
In doGet() parameters are not encrypted where as in doPost() method parameetrs are encrypted
doGet() is not suitable for file upload operation but doPost() is suitable for multiple part from data.
- Can you disable the caching on the back button of a particular browser?
Yes, The Caching process can be disabled on the back button of the browser.
The following tag is used for disable caching
<% response.setHeader("Cache-Control","no-store"); response.setHeader("Pragma","no-cache"); response.setHeader ("Expires", "0"); %>
- What are the methods available in session object?
The following methods are available in session object
- public Object getAttribute(String name)
- public Enumeration getAttributeNames()
- public long getCreationTime()
- public String getId()
- public long getLastAccessedTime()
- public int getMaxInactiveInterval()
- public void invalidate()
- public boolean isNew()
- public void removeAttribute(String name)
- public void setAttribute(String name, Object value)
- public void setMaxInactiveInterval(int interval)
- What is the role of Class.forName while loading drivers?
Class.forName creates an instance of JDBC driver and register with DriverManager. forName() is found in java.lang.Class class is used to get the instance of this Class with the specified class name.
Syntax:
public static Class<T> forName(String className) throws ClassNotFoundException
Sample Program:
public class InterviewTest {
public static void main(String[] args) throws ClassNotFoundException{
Class strcls = Class.forName("java.lang.String");
System.out.print(strcls.toString());
}
}
- Why “No suitable driver” error occurs?
No suitable driver” occurs when we are calling DriverManager.getConnection method,
Unable to load exact JDBC drivers before calling the getConnection method.It may be
invalid or wrong JDBC URL.
In other words This error occurs if JDBC is not able to find a suitable driver for the URL
format passed to the getConnection() method and if incorrect driver class name or more
typically the JDBC database URL passed is not properly constructed or incorrect permission
to access the driver jar files.
- What are the exceptions in JDBC?
The following exceptions in JDBC
- batchUpdate Exception
- Data Truncation
- SQL Exception
- SQL Warning
- Give steps to connect to the DB using JDBC?
DriverManager and DataSourse are the two types of connections to connecting database using JDBC
DriverManager:
It will load the driver class with the help of class.forName(driver class) and Class.forName().
Post Loading it will pass the control to DriverManager.
DriverManager.getConnection() will create the connection to access the database.
DataSource:
For DataSource, no need to use DriverManager with the help of JNDI. It will lookup the DataSource from Naming service server. DataSource.getConnection() method will return Connection object to the DB.
Boost Your Skills with Naresh IT's Advanced Java Online Training
If you’re aiming to master the intricacies of Advanced Java and be interview-ready, Naresh IT offers the perfect platform. Our comprehensive Advanced Java Online Training provides:
- Live instructor-led classes by industry experts.
- Hands-on practical experience with real-time projects.
- Complete course material covering servlets, JSP, JDBC, Spring, and Hibernate.
- 24/7 support for clearing doubts and assisting with assignments.
- Job-oriented training focused on interview preparation and placement assistance.
At Naresh IT, we not only teach you Advanced Java but also help you sharpen your problem-solving skills with interview-focused training sessions.
Stay tuned for the rest of the blog where we dive into more interview questions for all technologies
Ready to upskill? Enroll in Naresh IT's Advanced Java Online Training today and take your career to the next level!
----------------------------------------------------------------------------------------------------
For More Details About Full Stack Courses : Visit Here
Register For Free Demo on UpComing Batches : Click Here